An Introduction to Automated Editing
Macros are a terrific way to make the editing process faster and more repeatable, they do though have some limitations.
- There are times when the order of the editing steps are important.
- They have to be applied one at a time.
- You have to keep track of what has already been done and what yet still remains.
A Workflow, or a Process, or a Recipe, what ever you may be used to calling it (I prefer Workflow) can be viewed as a set of ordered steps. These steps would usually correspond to the actions that you would typically code up in a set of Macros. We will automate a Workflow that runs the right steps at the right time and records the editing progress on each of the images.
Let’s quickly go over how how capturing and running a “Workflow” is going to work before we dive in. We capture the code fragments that describe the editing process in the same way that we did for the macros. We can either copy and paste from the python console to generate a series of commands, or we could copy them from a working macro.
The steps in a workflow aren’t in fact very different than the Commander Macros that we described in the earlier part of this tutorial, the only real difference is they are an ordered set to be followed.
The way that we are going to use the automation tools is a bit different than using macros. When we want to use a macro we are running it on the image that is open in editor window. The automation tools on the other hand run on a directory of images, so we run it without an image being open in the editor window.
We mark the images that are ready to move to the next step of the workflow and then run the automation tools. The automated update looks at each image in the work directory, if it is marked for update, it gets updated to the next step, if it is not marked for update it is left alone.
The automated update will move the marked images to the next step of their assigned workflow essentially keeping track of the ‘book-keeping’ for us. A time management benefit is if there are several images requiring a filter that takes a bit of time to run, you can go do something else while they are running.
Automation Tool Implementation – In Broad Strokes
Let’s touch upon the main ideas that we are going to use to implement the automated workflow recording and execution tasks that we are talking about above.
- The pseudo code will be read and parsed into a data structure. Each pseudo code block (or step) will have a name and consist of commands and comments. Each workflow will have a name and will contain an ordered set of steps or pseudo code blocks.
- The automation flow will generate a list of images in a given directory. It will then step through that list checking whether an image is marked for update or not.
- If an image is marked for update, the automation flow will determine the name of the workflow and the name next step to perform on the image. The name of the ‘workflow’ and the ‘next step’ will be used to select a list of commands.
- The list of commands will be run in an ‘exec’ loop:
- The ‘current step’ and ‘next step’ for the image will be incremented and then saved with the image.
Architecture
We commented in the section on macros that good architectural choices can accomplish our goal without making the code overly complex. This argument is as compelling for automated updating of our images. The Architecture and Code for our automated updating tools will be very similar to the ones used for capturing and running the “Commander” macros.
Execution Model
As in our discussion of the Macros, we will be breaking the execution of our Automation Functions into three different categories:
- User Interface Functions
- Base Class and Functions
- Pseudo Code
We won’t rehash these topics but instead comment on the difference, which is the structure of the pseudo code. The organization of workflows as a set of steps where each step is a set of commands prompts us to organize the pseudo code in a similar manner, where we think of steps containing a sequence of commands, and workflows containing a sequence of steps. In order to reflect this additional level of hierarchy or containment, we will use an additional keyword in the workflow pseudo code.
Data Model
There are two special types of data for the automated flow:
- Trees and XML data are similar to the “Commander Macros”.
- Parasite (or Property) data to keep track of the particular workflow that is being used to edit an image, the next step that is to be used, and whether an image is ready to be incremented to the next step.
The Image and Parasites (or Property) Data
The Image type that we will be using for all of our work is the native Gimp *.xcf format. This image format saves all of the layers and modes that we might set while editing and also saves a type of data called Parasites, which are similar to Properties on many other systems.
Parasites are like variable can be referenced by name in order to access their value. Like variables they can be assigned and read. Unlike variables, parasites are persistent, very persistent. A parasite, when assigned to an image, becomes part of the image and is saved with the image file. A parasite that is assigned and saved with an image can be read after gimp is closed and reopened, it is just like any other file data in that respect.
Parasites are also very portable, you can read and write parasites using either the scheme based or python based scripts. They are also independent of the operating system, so you can write a parasite to an image on your Linux Desktop machine, and read it a week later on your windows based laptop assuming that you saved the images in the native gimp *.xcf file format. Parasites can also be written to specific layers, but for our present needs, the image parasites are all we are using.
Because the parasite is associated with the image, and it is persistent until it is overwritten or removed, it is an ideal tool for keeping track of the state of the image’s progress in the editing process. Beyond being able to just take notes, the values of the parasites can be used like a property to make decisions and influence the execution of the script that has read the parasite data.
If for example we opened an image that had two parasites (properties), named ‘UpdateFlag’ and ‘Flow’, we could use the values from those parasites to make decisions:
Example – Decisions based on Parasite / Property Values
UpdateFlag = str(theImage.parasite_find('UpdateFlag')) Flow = str(theImage.parasite_find('Flow')) if (UpdateFlag == 'YES'): if (Flow == 'Standard'): { run commands for Standard flow } elif (Flow == 'SemiAuto'): { run commands for SemiAuto Flow } elif (UpdateFlag == 'NO'): { do nothing }
Reading and writing parasites to an image does have one idiosyncrasy worth comment on which is the format of the data being written. You must express the parasite as an ordered set of ‘Name’, ‘Index’, and ‘Value’. Name and Value are both strings, and the Index is a small integer, (stay between 1 and 254). If you have not used parasites before you might be wondering how you determine a ‘correct’ value for the index. You may:
- Throw a dart at a dartboard and use the result (assuming you hit the board).
- Or, feel free to use my personal favorite ‘5’.
As long as you pick a number and preferably stick with it, everything will be fine. When you read the parasite value, the functions in the Scheme scripting interface will give you the ‘Name’, ‘Index’, and ‘Value’; the functions in the Python scripting interface will only return the ‘Name’ and ‘Value’.
Writing the parasite is called ‘attaching’ and reading the value back is called either ‘get’ or ‘find’ depending on the method you choose to use. You can read and write parasites from within scripts or from either of the python or scheme consoles.
Running the Automation Tools on a Workflow
Our final topic about an Automated Workflow in our “Broad Strokes” is how to setup and run the Workflow on a set of images. We are running on a set of images rather than just one so we open up gimp without an image in the editor window. The tools will open up, work on, save, and close the images one by one.
In an earlier tutorial “Automated Jpg to Xcf” we outlined how to import a directory containing jpeg images into a directory with gimp xcf images. The automation tool implementation modifies the import function to add the assignments of parasites to the images. The images are assigned to a particular flow as they are imported, and the initial set of flow control properties are written and saved as part of the import process. The jpeg-to-xcf function also runs the Automation Process (Auto Update Image) one time to put all of the images on the first step of the assigned flow, so they are ready for their first manual adjustment right after they are imported.
After opening and (optionally) adjusting an image you mark the image as ready for the next step by setting the “UpdateFlag” parasite to a value of “YES”. This is accomplished with a function that is available from the menu: “Automation → A2 Mark for AutoUpdate (File)”. (Note: Since you will be doing this a lot, it is very convenient to use a keyboard shortcut to run this function).
Images are moved to the next step in their flow by running the “Auto Update Images (Directory)” function from the menu. This will increment all of the images whose UpdateFlags are set to YES to the next step in their flow. Note that since each image has a record of its own next step and flow, there is no requirement for the images in a directory to be on the same step or even using the same workflow.
The mechanics for creating XML from pseudo code for the workflows, properties, and commander macros is to run the function Pseudocode to XML from the menu (Automation → Utilities → Pseudocode to XML).
The export XCF to JPG function in the “Automation” menu opens each xcf file in the source / work directory and looks at the properties of the image. If the image is “Finished”, at the end of the flow, it is exported. The images that are still being work on are left alone.
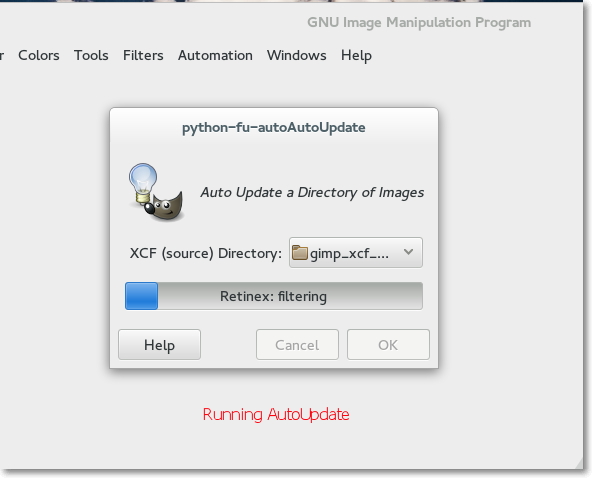
لا ترحل قبل إضافة تعليقك هنا - نحترم الانتقاد البناء والكلام السيء مردود على صاحبة
EmoticonEmoticon